[TypeScript] TypeScript に関する参考資料
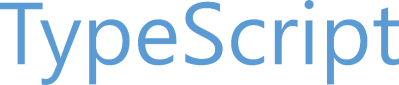
TypeScript に関する参考資料を集めてみた。
Web
- TypeScript
http://www.typescriptlang.org- TypeScript Language Specification Version 0.9.5 (2013/11/18)
http://www.typescriptlang.org/Content/TypeScript%20Language%20Specification.pdf - Tutorial
http://www.typescriptlang.org/tutorial/ - Playground
http://www.typescriptlang.org/Playground/
- TypeScript Language Specification Version 0.9.5 (2013/11/18)
- TypeScript - MSDN Blogs
http://blogs.msdn.com/b/typescript/ - TypeScript - CodePlex
https://typescript.codeplex.com/ - TypeScript for Visual Studio 2012 and 2013 - Microsoft Download Center (2013/12/5)
http://www.microsoft.com/en-us/download/details.aspx?id=34790 - MSDN マガジン
http://msdn.microsoft.com/ja-jp/magazine/- JavaScript - TypeScript: .NET 開発者の JavaScript への抵抗をなくす - MSDN マガジン January 2013 (2013/01)
http://msdn.microsoft.com/ja-jp/magazine/jj883955.aspx - 最新のアプリ - 最新のアプリで TypeScript を使用する - MSDN マガジン June 2013 (2013/06)
http://msdn.microsoft.com/ja-jp/magazine/dn201754.aspx
- JavaScript - TypeScript: .NET 開発者の JavaScript への抵抗をなくす - MSDN マガジン January 2013 (2013/01)
- Channel 9
http://channel9.msdn.com- Anders Hejlsberg: Introducing TypeScript (2012/10/01)
https://channel9.msdn.com/posts/Anders-Hejlsberg-Introducing-TypeScript - Anders Hejlsberg, Steve Lucco, and Luke Hoban: Inside TypeScript (2012/10/02)
http://channel9.msdn.com/posts/Anders-Hejlsberg-Steve-Lucco-and-Luke-Hoban-Inside-TypeScript - Anders Hejlsberg and Lars Bak: TypeScript, JavaScript, and Dart (2012/10/11)
http://channel9.msdn.com/Shows/Going+Deep/Anders-Hejlsberg-and-Lars-Bak-TypeScript-JavaScript-and-Dart - Introducing TypeScript: A language for application-scale JavaScript development (by Anders Hejlsberg) - Build 2012 (2012/11/01)
http://channel9.msdn.com/Events/Build/2012/3-012 - TouchDevelop: A touch-first IDE for the Web created with TypeScript - Build 2012 (2012/11/01)
http://channel9.msdn.com/Events/Build/2012/3-018 - Inside Typescript (by Charles Torre and Anders Hejlsberg) - Build 2012 (2012/11/02)
http://channel9.msdn.com/Events/Ch9Live/Channel-9-Live-at-BUILD-2012/Inside-Typescript - Introducing TypeScript (by Bart De Smet) - TechDays Belgium 2013 (2013/03/05)
http://channel9.msdn.com/Events/TechDays/Belgium-2013/20 - Anders Hejlsberg, Steve Lucco, Luke Hoban: TypeScript 0.9 - Generics and More (2013/06/18)
http://channel9.msdn.com/Blogs/Charles/Anders-Hejlsberg-Steve-Lucco-and-Luke-Hoban-Inside-TypeScript-09 - TypeScript: Application-Scale JavaScript (by Anders Hejlsberg and Steve Lucco) - Build 2013 (2013/06/26)
http://channel9.msdn.com/Events/Build/2013/3-314 - Anders Hejlsberg Q&A: TypeScript, C#, Roslyn, and More - Build 2013 (2013/06/27)
http://channel9.msdn.com/Events/Build/2013/9-006 - Getting started with TypeScript (by Johannes Rieken) - Visual Studio Online "Monaco" (2013/11/13)
http://channel9.msdn.com/Series/Visual-Studio-Online-Monaco/Getting-started-with-TypeScript - Introducing TypeScript (Preview) (by Jonathan Turner) - Visual Studio 2013 Launch (2013/11/13)
http://channel9.msdn.com/Events/Visual-Studio/Launch-2013/WC109
- Anders Hejlsberg: Introducing TypeScript (2012/10/01)
- TypeScript - Wikipedia
https://ja.wikipedia.org/wiki/TypeScript - TypeScript クイックガイド - phyzkit.net
http://phyzkit.net/typescript/ - TypeScript プログラミング - Solab
http://docs.solab.jp/typescript/ - 日本TypeScriptユーザー会
https://groups.google.com/forum/#!forum/typescript-japan - TypeScript - vvakameのブックマーク - はてなブックマーク
http://b.hatena.ne.jp/vvakame/TypeScript/ - @IT
http://www.atmarkit.co.jp- TypeScript(プレビュー版)概説(前編) : アンダース氏が設計した新言語による次世代JavaScript開発とは? - @IT (2013/01/18)
http://www.atmarkit.co.jp/ait/articles/1301/18/news087.html - TypeScript(プレビュー版)概説(後編) : 次世代JavaScript系言語「TypeScript」の主要言語機能 - @IT (2013/02/01)
http://www.atmarkit.co.jp/ait/articles/1302/01/news112.html - Microsoft、JavaScriptのスーパーセット「TypeScript」を発表 - @IT (2012/10/02)
http://www.atmarkit.co.jp/news/201210/02/typescript.html
- TypeScript(プレビュー版)概説(前編) : アンダース氏が設計した新言語による次世代JavaScript開発とは? - @IT (2013/01/18)
- 3日時間をもらったのでTypeScriptを触ってみた - slideshare (2013/10/15)
http://www.slideshare.net/y-kato/3-typescript - 部屋とボードゲームと私と酒と泪と男と女
https://sites.google.com/site/jun1sboardgames/- JavaScriptを書くならTypeScriptを使え! - (2012/11/03)
https://sites.google.com/site/jun1sboardgames/programming/typescript - TypeScriptで複数ファイルを扱おうとしてハマった点まとめ (2012/10/11)
https://sites.google.com/site/jun1sboardgames/programming/typescript_module - TypeScriptによるオブジェクト指向講座:ModelとView (2012/10/16)
https://sites.google.com/site/jun1sboardgames/programming/typescript_de_oo
- JavaScriptを書くならTypeScriptを使え! - (2012/11/03)
- neue cc
http://neue.cc- linq.jsのTypeScript対応とTypeScript雑感 (2012/10/12)
http://neue.cc/2012/10/12_382.html - 既存JavaScriptをTypeScriptとして修正する方法 (2012/10/21)
http://neue.cc/2012/10/21_385.html - TypeScript 0.9のジェネリクス対応でlinq.jsの型定義作って苦労した話 (2013/06/20)
http://neue.cc/2013/06/20_408.html
- linq.jsのTypeScript対応とTypeScript雑感 (2012/10/12)
- TypeScript Keynote (by Anders Hejlsberg) - GOTO Conferences - YouTube (2012/10/07)
https://www.youtube.com/watch?v=3dqZW_DqHIQ - TypeScriptの基礎から実践・利用事例まで - 第1回 Build Insider OFFLINE (2013/06/08)
http://www.buildinsider.net/hub/bioff/o2
書籍
- TypeScript For JavaScript Programmers (by Steve Fenton)
- ペーパーバック (2012/10/29)
http://www.amazon.co.jp/dp/1291107371/ - Kindle版 (2012/10/01)
http://www.amazon.co.jp/dp/B009KWNA7O/
- ペーパーバック (2012/10/29)
- TypeScript Revealed (by Dan Maharry)
- ペーパーバック (2013/01/30)
http://www.amazon.co.jp/dp/1430257253/ - Kindle版 (2013/01/28)
http://www.amazon.co.jp/dp/B00ACC6C26/
- ペーパーバック (2013/01/30)
- TypeScript for C# Programmers (bySteve Fenton)
- ペーパーバック (2013/10/12)
http://www.amazon.co.jp/dp/1291576215/
- ペーパーバック (2013/10/12)